Subscribe to updates
Writing a Compiler in TypeScript
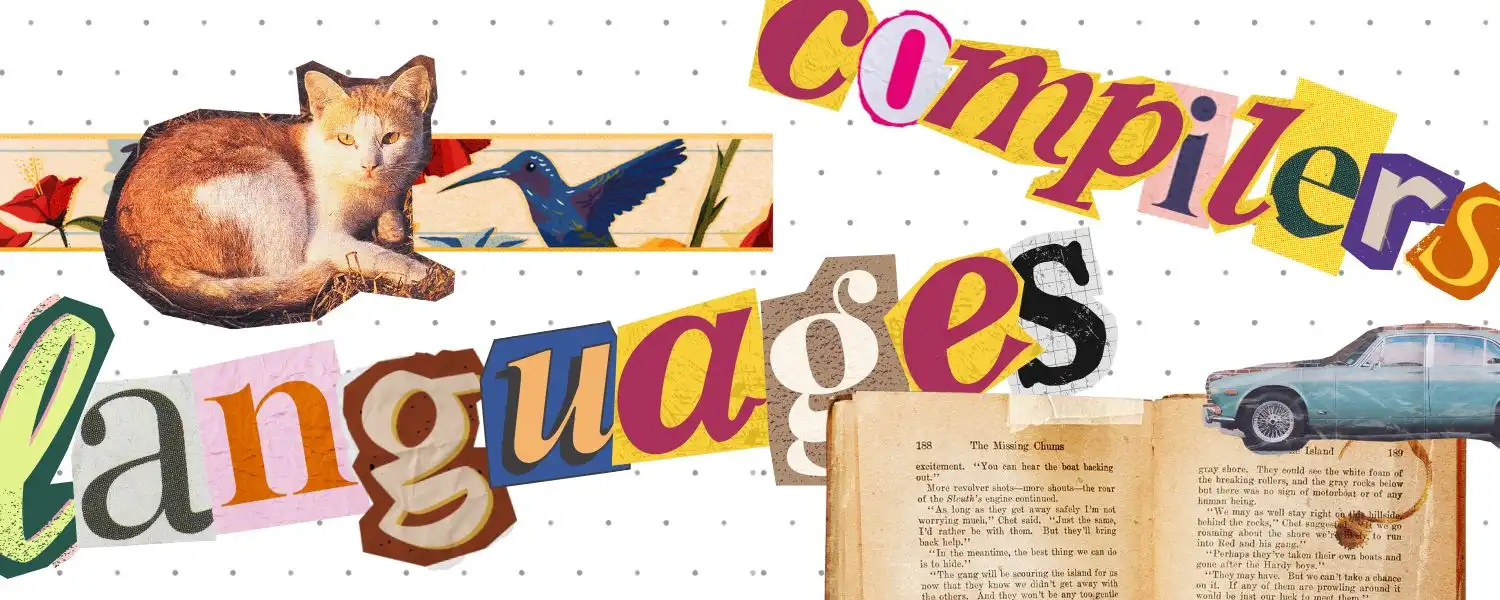
Compilers! Aren’t they fascinating? If you’ve ever thought about how they worked and about building one, welcome! These set of pages are for you. We’ll be building a compiler from scratch for a toy language called Gom - a language with a simple, familiar syntax.
import io;
fn main() { io.log("Hello, World!");}
What will we be building?
We’ll first introduce Gom, a simple language that we’ll build a compiler for. Compilers are programs that understand some text and convert it into another form, usually a lower-level language. In our case, we’ll be converting Gom code into LLVM intermediate representation (IR), which is a platform-independent representation of code that can then be compiled to machine code. Simply put, our compiler will convert Gom code into executable machine code.
In our journey, we’ll go through various phases of a compiler:
- Lexical Analysis: Make sense of the text and convert it into Gom-tokens
- Parsing: Read the tokens in their order and make sense of the structure of the code
- Semantic Analysis: Understand the meaning of the code and check for errors, e.g. type mismatches
- Code Generation: Convert the AST into LLVM IR
We’ll approach each topic directly with code to remove any fluff and to help you understand the concepts quickly. Well, writing a compiler isn’t that hard honestly! The hard parts are in the details, and you can go as deep as you want. The initial four chapters cover what you need to implement a basic compiler, something that would give you your own toy language in the end.
If you like the journey, you can decide to then explore the advanced chapters (Chapter 5 and beyond) that cover more complex topics like type systems, optimizations, and more.
Why TypeScript?
You might ask this, given that TypeScript is a rare choice in production compilers. Well, while learning a new concept (which may have it’s own complexities), it’s best to use a language that you are comfortable with. TypeScript is my go-to language at work and side projects, and that’s how I started writing the Gom compiler. For all fellow TypeScript users, this course aims to teach you compiler construction in a language you are already familiar with.
Phil Eaton quotes a beautiful message that talks about avoiding the confusion of choosing the “best language” for writing databases. The same applies to compilers!
Course Updates
I am still writing the course and am releasing chapters as they are ready. If you’d like to be notified when new content is available, you can subscribe to the email list below. I promise not to spam you, and you can unsubscribe anytime.
Know more about me and read my blog here: https://mohitkarekar.com/